In the fast-paced world of web development, staying current with the latest technologies is key to ensuring your website runs efficiently and securely. PHP 8.0 is here, and it brings exciting features and performance enhancements that can elevate your website to the next level. In this DIY guide, we’ll walk you through the process of checking and migrating your site to PHP 8.0, making the transition smooth and accessible for enthusiasts like you.
Why Upgrade to PHP 8.0?
Let’s start by exploring why upgrading to PHP 8.0 is beneficial. From performance boosts to new capabilities, PHP 8.0 is designed to enhance your website’s functionality and improve developer productivity. We’ll delve into these advantages to help you understand why this upgrade is worth your time and effort.
Understanding Compatibility
Upgrading to a new PHP version can be an exciting venture, but it’s crucial to understand compatibility to ensure a smooth transition. Here, we’ll delve deeper into the key aspects of compatibility to ease any concerns you might have.
Backward Compatibility Explained:
One of the foremost concerns when migrating to PHP 8.0 is the potential impact on existing code. The good news is that PHP 8.0 strives to maintain backward compatibility with previous versions, including PHP 7.4. Backward compatibility ensures that your existing codebase, written in earlier PHP versions, should continue to function correctly.
However, it’s essential to be aware that PHP 8.0 does introduce some changes and deprecations. Deprecations are features or functions that are discouraged and will likely be removed in future PHP versions. The PHP community provides migration guides that detail these changes, helping you identify and update any deprecated code.
Navigating Deprecated Features:
To facilitate a smooth transition, it’s crucial to identify and address any deprecated features in your codebase. Deprecated features are marked for removal in future PHP versions, and while they might still work in PHP 8.0, it’s recommended to update your code to use alternative, non-deprecated features.
Common examples of deprecated features include outdated functions or methods that have newer, more efficient alternatives. For instance, certain functions might have undergone changes in their usage or have been replaced with improved counterparts. Our guide will walk you through specific examples and how to update your code accordingly.
Breaking Changes and Potential Stumbling Blocks:
While PHP 8.0 aims for backward compatibility, there are instances where certain changes might lead to unintended consequences in your code. These changes could be related to stricter type checking, altered behavior of certain functions, or adjustments in error handling.
Our guide will help you identify potential stumbling blocks and provide practical solutions to address these issues. Whether it’s adjusting type hints, updating error handling mechanisms, or refactoring specific parts of your code, we’ve got you covered with actionable steps.
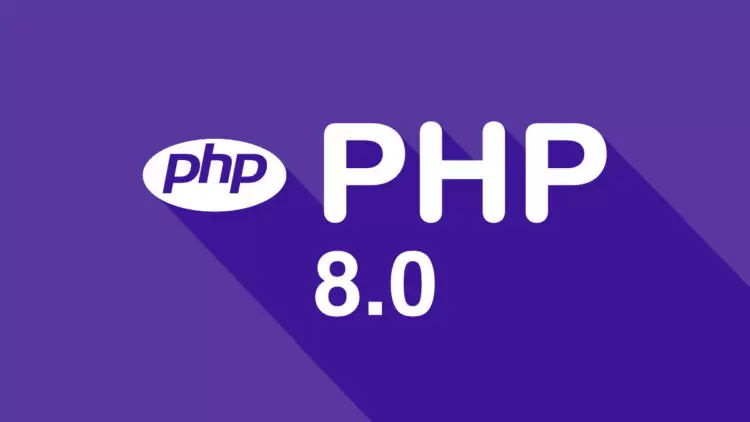
Proactive Measures for Compatibility:
To stay ahead of compatibility issues, we’ll guide you on adopting proactive measures. This includes leveraging PHP’s built-in tools for identifying deprecated features within your codebase before initiating the migration process. By proactively addressing these issues, you can ensure a smoother transition to PHP 8.0.
In summary, understanding compatibility involves navigating backward compatibility, addressing deprecated features, anticipating potential stumbling blocks, and taking proactive measures. Armed with this knowledge, you’ll approach the migration process with confidence, knowing how to handle existing code and make the necessary adjustments for a successful transition to PHP 8.0.
Assessing Your Current Environment
Before diving into the migration process, let’s ensure you know your starting point. We’ll walk you through checking your current PHP version, providing step-by-step instructions tailored to various hosting platforms.
For cPanel Users:
- Log in to cPanel.
- Locate the “PHP Selector” or “Select PHP Version” option.
- View your current PHP version.
For Plesk Users:
- Access Plesk Control Panel.
- Navigate to “PHP Settings.”
- Check your PHP version.
For Shared Hosting with Custom Control Panel:
- Log in to your hosting control panel.
- Locate “PHP” or “Server Settings.”
- Find PHP version information
For VPS/Dedicated Server Users:
- Access the server via SSH.
- Run the PHP version command.
Section 4: Compatibility Check Tools
No need to manually inspect every line of code! We’ll introduce you to handy tools that automate the compatibility checking process. From PHP Compatibility Checker to other static analyzers, we’ll help you choose the right tools for your DIY toolkit.
1. PHP Compatibility Checker:
Installation:
Start by installing the PHP Compatibility Checker on your local development environment or staging server. You can typically do this through the command line using a tool like Composer.
How to Use:
Run the tool against your codebase. Open your command line, navigate to your project’s main folder, and run the compatibility checker command.
phpcs --standard=PHPCompatibility --runtime-set testVersion 8.0-8.0 your_project_directory
Understanding the Results:
Carefully review the generated report. The tool will point out any deprecated functions or syntax that’s incompatible with PHP 8.0.
Fixing Issues:
Use the information in the report to make necessary changes in your code. The tool often suggests alternative approaches for deprecated functions.
2. Static Analyzers (e.g., PHPStan, Psalm):
Installation:
- Choose a static analyzer like PHPStan or Psalm based on your preference.
- Install your chosen tool using Composer:
composer require --dev phpstan/phpstan
or
composer require --dev vimeo/psalm
Configuration:
Set up the tool to work with your project’s structure. You might need to create a configuration file (e.g., phpstan.neon or psalm.xml).
Running the Analyzer:
Run the analyzer from your command line. Navigate to your project’s main folder and use these commands:
vendor/bin/phpstan analyse
or
vendor/bin/psalm
Reviewing the Results:
The tool will provide insights into potential issues within your codebase.
Fixing Issues:
Go through the report and address any problems you find. These tools often offer detailed explanations and suggestions for solutions.
3. Online Compatibility Checkers:
Choosing a Platform:
Select an online compatibility checker, such as “PHP Compatibility Test,” or a similar platform.
Uploading Code or Providing a Repository URL:
Depending on the tool, either upload your codebase or provide the URL of your repository.
Reviewing the Results:
The online checker will generate a compatibility report, usually highlighting specific files and lines with potential issues.
Taking Action:
Use the provided information to make the necessary adjustments to your code.
These tools are like your helpful companions, making sure you catch compatibility issues early in the process. Armed with their insights, you’ll be well-prepared to ensure a smooth transition to PHP 8.0.
Handling Deprecated Features
Not sure what deprecated features are and how they impact your code? We’ve got you covered with examples of commonly deprecated features in PHP 8.0 and clear instructions on how to update them.
Example:
// Deprecated feature in PHP 7.4 $deprecatedVar = $someObject->$someDeprecatedMethod(); // Updated code for PHP 8.0 $updatedVar = $someObject->{$someUpdatedMethod}();
Testing Your Website Locally
To ensure a smooth migration, it’s crucial to test your website in a controlled environment. We’ll guide you through setting up a local development environment, installing PHP 8.0, and running a copy of your website for testing purposes.
Creating a Backup Plan
Before making any changes, we’ll emphasize the importance of creating backups. We’ll discuss various backup methods, ensuring you have a safety net in case anything goes awry during the migration.
Addressing Common Migration Challenges
Expect the unexpected! We’ll discuss common challenges DIYers might face during migration and provide practical solutions. Whether it’s incompatible extensions or deprecated functions, we’ll help you navigate through potential roadblocks.
Leveraging New Features of PHP 8.0
Once your website is successfully migrated, we’ll highlight the exciting features PHP 8.0 brings to the table. We’ll provide simple examples to help you leverage named arguments, union types, and match expressions in your code.
Named Arguments Example:
// Before PHP 8.0 function setPersonDetails($name, $age, $city) { // Function logic } setPersonDetails('John', 25, 'New York'); // Positional arguments // In PHP 8.0 function setPersonDetailsWithNamedArgs($name, $age, $city) { // Function logic } setPersonDetailsWithNamedArgs(name: 'John', age: 25, city: 'New York'); // Named arguments
Union Types Example:
// Before PHP 8.0 function processValue($value) { if (is_string($value)) { // Handle string } elseif (is_int($value)) { // Handle integer } } // In PHP 8.0 function processValueWithUnionType(string|int $value) { // You can confidently use $value as either a string or an integer }
Match Expressions Example:
// Before PHP 8.0 function getStatus($status) { switch ($status) { case 'success': return 'Operation was successful'; case 'error': return 'An error occurred'; default: return 'Unknown status'; } } // In PHP 8.0 function getStatusWithMatchExpression($status) { return match($status) { 'success' => 'Operation was successful', 'error' => 'An error occurred', default => 'Unknown status', }; }
Final Testing and Deployment
Before the big reveal, we’ll guide you through the final testing phase. From functional tests to performance checks, we’ll ensure your website is ready for prime time. Then, we’ll walk you through the deployment process, whether you’re on a shared hosting platform or a VPS.
In conclusion, upgrading to PHP 8.0 is a rewarding journey that can significantly enhance your website’s performance and capabilities. We’ve covered everything from compatibility checks to leveraging new features, empowering you to take charge of your site’s future. Embrace the upgrade, share your success stories, and feel free to ask questions in the comments – the DIY community is here to support you.
Additional Resources and Next Steps
For those eager to dive deeper, we’ve compiled a list of additional resources, including links to the official PHP documentation and online communities where you can seek further assistance. Your journey doesn’t end here; there’s always more to explore and learn in the ever-evolving world of web development.
As you navigate through the intricacies of PHP migration, remember that expert assistance is just a click away. If you’re encountering roadblocks, looking to speed up the process, or seeking personalized guidance, consider tapping into the expertise of skilled PHP developers available on Codeable.
Visit Codeable now and connect with experienced developers who can provide hands-on support and solutions tailored to your specific needs.
Don’t let challenges slow you down – empower your migration journey with Codeable’s expert assistance.
Upgrading to PHP 8.0 FAQ
1. What are the key features introduced in PHP 8.0?
PHP 8.0 introduces features like the JIT compiler, named arguments, union types, attributes, and match expression.
2. Is PHP 8.0 backward compatible with code written in PHP 7.4?
PHP 8.0 aims for backward compatibility, but some changes exist. Review migration guides and update deprecated features for compatibility.
3. How does the Just-In-Time (JIT) compiler in PHP 8.0 improve performance?
The JIT compiler translates PHP bytecode into machine code, improving performance for CPU-intensive tasks. However, the impact depends on the application.
4. What is the significance of named arguments, and how do they differ from positional arguments?
Named arguments allow passing values based on parameter names, improving code readability. This eliminates the need to remember parameter order.
5. How can developers leverage union types introduced in PHP 8.0?
Union types allow declaring that a variable can hold values of multiple types, enhancing type flexibility and providing expressive type hints.
6. What are attributes in PHP 8.0, and how do they differ from docblocks?
Attributes are a structured way to add metadata, replacing docblocks in many cases. They offer better tooling support and compile-time validation.
7. Are there any changes to error handling in PHP 8.0?
Yes, PHP 8.0 introduces the throw expression, simplifying error handling by allowing exceptions to be thrown in expressions.
8. How can developers address deprecations and removals of features in PHP 8.0?
Review migration guides, identify deprecated features, and promptly address removals to ensure compatibility with future PHP versions.
9. What is the match expression, and how does it differ from the switch statement?
The match expression is a concise replacement for the switch statement, supporting value comparisons and providing a more consistent syntax.
10. How can developers effectively test their code during the migration process?
Comprehensive testing is essential. Use unit tests, integration tests, and tools like static analyzers to catch potential issues. Run the application in a PHP 8.0 environment for runtime error identification