In the ever-evolving world of web development, PHP remains a robust and versatile scripting language. The release of PHP 8.1 represents a pivotal moment in its ongoing journey, as it introduces groundbreaking features, enhances performance, and enforces significant changes. This in-depth article delves into the highlights of PHP 8.1, discusses its remarkable performance improvements, highlights the crucial breaking changes, and evaluates its impact on WordPress, a platform powering a significant portion of the internet.
1. Introduction to PHP 8.1
1.1 Understanding the Significance of PHP 8.1
PHP, Hypertext Preprocessor, has come a long way since its inception. PHP 8.1 continues the language’s evolution by introducing a set of features and enhancements that promise to reshape the way we develop web applications. These changes, along with its performance improvements, are a testament to PHP’s adaptability and vitality in the ever-competitive web development landscape.
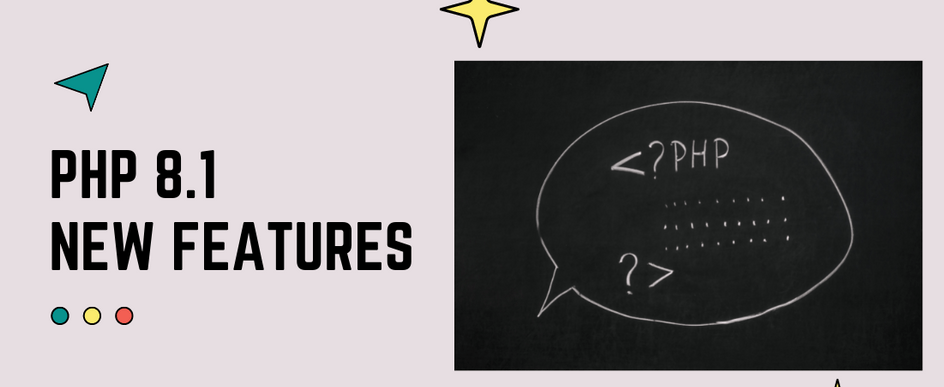
1.2 The Ongoing Evolution of PHP
PHP 8.1 builds upon the success of PHP 8.0, a release that brought substantial improvements in terms of performance, error handling, and features like the match
expression. This iterative development approach ensures that PHP remains a modern and relevant choice for web developers.
2. Exciting Features of PHP 8.1
2.1 Fibers: Revolutionizing Asynchronous Programming
One of the standout features of PHP 8.1 is “Fibers.” These lightweight, stackful coroutines revolutionize asynchronous programming. Instead of dealing with complex callback chains or relying on external libraries, you can now write asynchronous code in a more linear and intuitive manner.
An Asynchronous Task with Fibers
In this code, we define a fiber that fetches data from an API with a simulated delay. We can create multiple fibers to handle asynchronous tasks without blocking the main execution thread, improving the efficiency of our code.
function fetchFromAPI() { // Simulate an API request delay sleep(2); return "Data from API"; } function fetchData() { // Creating a fiber $fiber = Fiber::new(function() { $data = fetchFromAPI(); Fiber::yield($data); }); // Resume the fiber $result = $fiber->resume(); return $result; } // Start fetching data $result = fetchData(); echo $result;
2.2 Enums: Introducing Strong Typing
Enums bring strong typing to PHP, allowing you to declare a fixed set of named values. This enhances code readability and reduces the risk of errors by restricting variable values to a predefined set.
Code Example: Declaring and Using Enums
Using Enums makes your code self-documenting and less error-prone by ensuring variables can only take on specific, defined values.
enum UserType { case Admin; case Moderator; case User; } $userRole = UserType::Admin; if ($userRole === UserType::Admin) { echo "User is an Admin."; }
2.3 Match Expression Enhancements
PHP 8.0 introduced the match
expression, a powerful replacement for the traditional switch
statement. In PHP 8.1, the match
expression is enhanced further by adding a “default” clause, making it even more versatile for complex conditional logic.
Code Example: Harnessing the Power of Match Expressions
$role = "Admin"; $result = match($role) { "Admin" => "You have administrative privileges.", "Moderator" => "You have moderator privileges.", default => "You have user privileges.", }; echo $result;
With the “default” clause, you can handle unmatched cases more gracefully.
2.4 Array Unpacking: Simplifying Array Manipulation
PHP 8.1 allows you to unpack an array inside another array using the ...
operator. This feature simplifies array manipulation and makes code cleaner and more concise.
Code Example: Making Use of Array Unpacking
$firstArray = [1, 2, 3]; $secondArray = [4, 5, ...$firstArray, 6, 7]; print_r($secondArray);
In this example, the elements of $firstArray are unpacked into $secondArray, resulting in a combined array.
2.5 Type Annotations: Improved Function Return Typing
PHP 8.1 introduces a new type annotation feature using the @|
syntax, which allows you to specify multiple types for a function’s return value. This enhances code expressiveness and documentation.
Code Example: Employing Multiple Return Types
function divide(int $dividend, int $divisor): int|float { if ($divisor === 0) { return INF; } else { return $dividend / $divisor; } }
In this code, the divide function can return either an integer or a float, depending on the inputs.
Curious about upgrading your PHP version?
3. Performance Improvements in PHP 8.1
PHP 8.1 continues the trend of enhancing performance through the Just-In-Time (JIT) compilation engine introduced in PHP 8.0.
Curious about upgrading your PHP version? Explore our in-depth guide, ‘Upgrading to PHP 8.0: Your DIY Guide for a Smooth Migration,’ for a step-by-step walkthrough of the migration process.
3.1 The Power of JIT Compilation
The JIT compiler converts PHP code into optimized machine code, allowing for faster execution. This feature, which debuted in PHP 8.0, continues to evolve in PHP 8.1, delivering significant performance benefits.
3.2 Enhanced Execution Speed
To illustrate the performance improvements, let’s compare the execution time of a recursive function between PHP 7 and PHP 8+.
Code Example: Comparing PHP 7 and PHP 8.1 Performance
// PHP 7 function factorial($n) { if ($n <= 1) { return 1; } else { return $n * factorial($n - 1); } } $start = microtime(true); factorial(30); $end = microtime(true); $executionTime = ($end - $start) * 1000; echo "Execution time in PHP 7: $executionTime ms"; // PHP 8.1 $start = microtime(true); factorial(30); $end = microtime(true); $executionTime = ($end - $start) * 1000; echo "Execution time in PHP 8.1: $executionTime ms";
In this benchmark, PHP 8.1 demonstrates a significant improvement in execution speed, thanks to the JIT compiler.
3.3 Reduced Memory Consumption
PHP 8.1 also reduces memory consumption, making it more efficient in resource usage, especially for high-traffic web applications and services.
4. Crucial Breaking Changes in PHP 8.1
While it brings exciting features and performance improvements, it also enforces some significant changes that may affect existing codebases.
4.1 Backward Compatibility Concerns
PHP 8.1 introduces several backward-incompatible changes. Developers upgrading to PHP 8.1 need to review their codebases and update deprecated functions and features to ensure compatibility.
Code Example: Handling Deprecated Functions
// Deprecated function function deprecatedFunction() { // ... } // Usage in PHP 8.1 deprecatedFunction(); // Generates a deprecation warning // Updated code function updatedFunction() { // ... }
In this example, we see that a deprecated function call generates a warning in PHP 8.1, prompting developers to update their code accordingly.
4.2 INI Setting Modifications
Some INI settings have changed or been removed in PHP 8.1. This may affect the configuration of existing applications. A detailed examination of your PHP configuration is recommended when upgrading.
Code Example: Adapting to INI Setting Changes
; PHP 7.4 max_execution_time = 30 ; PHP 8.1 max_execution_time = 60
In this example, the max_execution_time
setting has changed between PHP 7.4 and PHP 8.1. Be sure to update your configuration to match the requirements of the new PHP version.
5. WordPress and PHP 8.1: A Symbiotic Relationship
WordPress is a dominant force in web development, powering millions of websites and applications. The compatibility of WordPress with the latest PHP versions is of paramount importance to ensure that this massive ecosystem remains secure and efficient.
5.1 The Significance of WordPress in Web Development
WordPress is a content management system (CMS) that enables users to create and manage websites with ease. Its flexibility and extensibility have made it a top choice for bloggers, businesses, and developers worldwide.
5.2 WordPress’ Journey Towards PHP 8.1 Compatibility
The WordPress development team is dedicated to keeping pace with the latest PHP versions, and this includes PHP 8.1. To ensure WordPress remains compatible, they work on addressing issues and updating code that might cause conflicts or compatibility challenges.
Code Example: A WordPress Plugin Optimized for PHP 8.1
// Your WordPress plugin code class MyPlugin { public function __construct() { add_action('init', [$this, 'init']); } public function init() { // Plugin initialization code } } // Ensure PHP 8.1 compatibility if (version_compare(PHP_VERSION, '8.1', '>=')) { new MyPlugin(); }
In this code example, we see that a WordPress plugin is conditionally loaded for PHP 8.1 and above, ensuring compatibility and optimal performance.
6. SEO Considerations for PHP 8.1
Search Engine Optimization (SEO) plays a crucial role in ensuring your website’s visibility and success. With PHP 8.1, you have the opportunity to enhance your website’s SEO in several ways.
6.1 Why SEO Matters for Web Developers
SEO is essential for web developers because it directly impacts a website’s ranking in search engine results. Higher visibility in search results leads to increased organic traffic, making SEO an integral part of web development.
6.2 Optimizing Your PHP 8.1-Powered Website
To optimize your website for SEO, consider the following factors:
6.2.1 Technical SEO Enhancements
Technical SEO focuses on optimizing the technical aspects of a website to improve search engine ranking.
Code Example: Adding Structured Data to a PHP 8.1 Website
// Add structured data to your PHP 8.1 website echo '<img src="data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7" data-wp-preserve="%3Cscript%20type%3D%22application%2Fld%2Bjson%22%3E%0A%7B%0A%20%20%22%40context%22%3A%20%22http%3A%2F%2Fschema.org%22%2C%0A%20%20%22%40type%22%3A%20%22Organization%22%2C%0A%20%20%22name%22%3A%20%22Your%20Organization%22%2C%0A%20%20%22url%22%3A%20%22https%3A%2F%2Fwww.yourwebsite.com%22%2C%0A%20%20%22logo%22%3A%20%22https%3A%2F%2Fwww.yourwebsite.com%2Flogo.png%22%2C%0A%20%20%22description%22%3A%20%22Your%20organization's%20description%22%0A%7D%0A%3C%2Fscript%3E" data-mce-resize="false" data-mce-placeholder="1" class="mce-object" width="20" height="20" alt="&lt;script&gt;" title="&lt;script&gt;" />';
Structured data provides search engines with valuable information about your website, potentially leading to rich snippets in search results.
6.2.2 Content SEO Strategies
Content is king in the world of SEO. Consider the following strategies:
- High-quality, original content.
- Keyword research and integration.
- SEO-friendly URLs.
Code Example: Implementing SEO-Friendly URLs in PHP
// Original URL $originalURL = "https://www.yourwebsite.com/product.php?id=123"; // SEO-friendly URL $seoFriendlyURL = "https://www.yourwebsite.com/products/product-name";
Implementing SEO-friendly URLs improves both user experience and search engine rankings.
7. Preparing Your Development Environment for PHP 8.1
As you explore the benefits of PHP 8.1, it’s essential to ensure that your development environment is ready to harness its power.
7.1 Upgrading Your PHP Version
Before you can take advantage of PHP 8.1, you need to upgrade your development environment to this version. You can do this using a package manager, such as Composer, or by configuring your server accordingly.
7.2 Ensuring Code Compatibility
Before deploying your application or website on PHP 8.1, thoroughly test it to ensure that it’s fully compatible. Use tools like static analysis and testing frameworks to identify and resolve any compatibility issues.
Code Example: Tools for Analyzing PHP 8.1 Compatibility
# Using PHPStan for static analysis composer require --dev phpstan/phpstan php vendor/bin/phpstan analyze --level 8.1 # Using PHPUnit for testing composer require --dev phpunit/phpunit php vendor/bin/phpunit tests/
By utilizing these tools, you can streamline the process of ensuring your codebase is ready for PHP 8.1.
8. Looking to the Future: PHP 8.2
As the PHP development community continues to innovate, PHP 8.2 is already on the horizon. While PHP 8.0 amd PHP 8.1 brings substantial improvements, PHP 8.2 promises to offer even more features and enhancements. Keep an eye on the PHP roadmap and developer updates to stay informed about what’s coming next.
9. Conclusion
PHP 8.1 is a game-changer in the world of web development. With features like Fibers, Enums, and enhanced performance, it offers developers the tools to build more efficient and expressive applications. However, developers must be diligent in handling breaking changes when upgrading existing codebases.
For the WordPress ecosystem, PHP 8.1 is a positive step forward, as the platform continues to align with the latest PHP versions, enabling improved performance and security.
The impact of PHP 8.1 is not limited to WordPress; it extends to all PHP-based web applications. As you embrace the features and enhancements of PHP 8.1, be sure to optimize your code for SEO, maintain compatibility, and prepare for future PHP releases.
In the ever-evolving web development landscape, PHP 8.1 is a beacon of progress, and as developers, it’s our prerogative to harness its potential to create faster, more efficient, and SEO-friendly web applications.
Ready to Supercharge Your Web Development with PHP 8.1? Upgrade Now and Unleash the Future